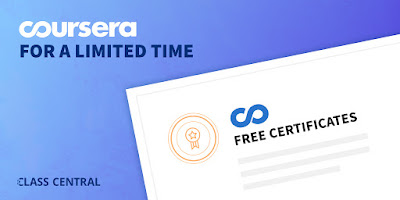
Syllabus
-How to convert an existing C program to C++. Use of type safe Input/Output.
Avoiding the preprocessor.
Module 2
-Review of Dijkstra's shortest path algorithm. C++ Functions and Generics. C++ classes and OO.
Point as an example.
Module 3
-Point: Default constructor and initializing syntax. Conversion Constructors. Copy Constructor. List and dynamic memory allocation. Deep Copy.
Module 4
-Prim’s and Kruskal’s algorithms. Use of basic Container Classes. Tripod-Container, Iterator, Algorithm.
Final Exam
-Practice and final.
Exam Practice 1
Question 1
FOR
ALL QUESTIONS IN THIS PRACTICE EXAM REFER TO THE FOLLOWING:
//What does this program
print?
#include <iostream>
using namespace std;
#define foo1( a ) (a * a)
inline int foo2( int a )
{ return (a * a); }
inline int goo1( int x )
{ return foo1(foo2(x)); }
inline int goo2( int&
x ) { return x = foo2(foo1(x)); }
inline int goo3( int&
x, int y ) { return foo2(foo1(y + 1)); }
inline void goo4(
int& x, int y ) { x = foo1(foo2(y + 1)); }
int main()
{
int i = 2, j = 1;
cout << "foo1
= " << foo1( 1 + 2 ) << "\n";
cout << "foo2
= " << foo2( 2 + 1 ) << "\n";
cout << "goo1
= " << goo1( i ) << "\n";
cout << "goo2
= " << goo2( j ) << "\n";
cout << "goo3
= " << goo3( i, j ) << "\n";
goo4( i, j );
cout << "i =
" << i << "\nj = " << j <<
"\n";
return 0;
}
Enter
the numerical answer forfoo1.
5
Question 2
Enter
the numerical answer for foo2.
9
3.Question 3
Enter
the numerical answer for goo1.
16
Question 4
Enter
the numerical answer for goo2.
1
Question 5
Enter
the numerical answer for goo3.
9
Question 6
Enter
the numerical answer for i.
16
Question 7
Enter
the numerical answer for j.
1
Final Exam
Question 1
FOR
QUESTIONS 1-5 REFER TO THE FOLLOWING VARIABLES AND INITIALIZATIONS:
int a = 2, b = -3, c = 2;
bool tval1 = false, tval2
= true;
char ch = ‘b’;
Assume each evaluation is
legal and occurs immediately after this declaration.
What is the value of the
following expression?
b
+ c * a
1
Question 2
What is the value of the
following expression? (Refer to question 1.)
c
% 5/2
1
Question 3
What is the value of the
following expression? (Refer to question 1.)
-a
* c++
-4
Question 4
What is the value of the
following expression? (Refer to question 1.)
tval1
&& tval2
0
Question 5
What is the value of the
following expression? (Refer to question 1.)
ch
+= 2
1
Question 6
What
did D. Ritchie do?
All of above
Question 7
What
did B. Stroustrup do?
Invented c++
Question 8
What
did E. Dijkstra invent?
Shortest path algoritham
Question 9
What
did L. Euler invent?
Graph theroy
Question
10
printin is a keyword in
C++11.
false
Question
11
In
the expression f(1) || (!g(2)), and without knowing the return types of f(1)
and g(2), you can still assert that f(1) will always be evaluated before g(2)
(which may not get evaluated at all).
true
Question
12
FOR
QUESTIONS 12–16 REFER TO THE FOLLOWING:
Answer number that is
returned by mystery() for each answer in printout.
//templates what gets
printed
#include <iostream>
using namespace std;
template <class T1,
class T2>
int mystery(T1& a, T2
b, int c)
{
T1 t = a;
a = a + b;
return ( a - t + c);
}
int main(void)
{
int a = 3;
double b = 2.5;
int c = 1;
cout << "
answer 1 is " << mystery(a, 2, c) << endl;
cout << "
answer 2 is " << mystery(a, 1, c) << endl;
a = 5;
cout << "
answer 3 is " << mystery(a, b, c) << endl;
a = 2;
b = 2.5;
cout << "
answer 4 is " << mystery(a, b, b) << endl;
cout << "
answer 5 is " << mystery(a,mystery(a, b, b),c)<< endl;
return 0;
}
Answer
1 is:
3
Question
13
Answer
2 is:
2
Question
14
Answer
3 is:
3
Question
15
Answer
4 is:
4
Question
16
Answer
5 is:
5
Question
17
FOR
QUESTIONS 17–19 REFER TO THIS WEIGHTED UNDIRECTED GRAPH
What
is the cost of its minimum spanning tree?
33
Question 18
What is the cost of its
maximum spanning tree? (A maximum weighted spanning tree is a spanning tree
whose edges add up to a max for all possible spanning trees.)
(Refer
to the graph in question 17.)
30
Question
19
What is the shortest path
cost from node 0 to node 2?
(Refer
to the graph in question 17.)
9
20.Question 20
FOR
QUESTIONS 20–24 REFER TO THE FOLLOWING:
//What does the following
print
#include <iostream>
#include <vector>
#include
<algorithm>
using namespace std;
int main()
{
vector<int>
data(5,1);
int sum {0};
cout << sum
<< endl;
for(auto element : data)
sum += element;
cout << sum
<< endl;
for(auto p =
++data.begin(); p != --data.end(); ++p)
sum += *p;
cout << sum
<< endl;
sum = 0;
data.push_back(2);
data.push_back(3);
for(auto element : data)
sum += element;
cout << sum
<< endl;
cout <<
accumulate(data.begin(), data.end(), data[0]) << endl;
}
What
does the first line print?
0
Question
21
What
does the second line print? (Refer to question 20.)
5
Question
22
What
does the third line print? (Refer to question 20.)
8
Question
23
What
does the fourth line print? (Refer to question 20.)
10
Question
24
What
does the fifth line print?
11
No comments:
Post a Comment
if you have any doubt please let me know